Seating
How to Render a Screen Layout
The following sections define how a screen layout should be rendered.
Screen and Seat Area Boundaries
{
"boundaryRight": 75,
"boundaryLeft": 25,
"boundaryTop": 45,
"screenStart": 30,
"screenWidth": 40,
…
}
The boundaryRight, boundaryLeft, boundaryTop properties define the entire area that the seats cover within the layout. These values are percentages (0 – 100%). The screenStart and screenWidth properties define how the screen is rendered in relation to the seats. The following image shows how the seat area (in purple) and the screen will be rendered:
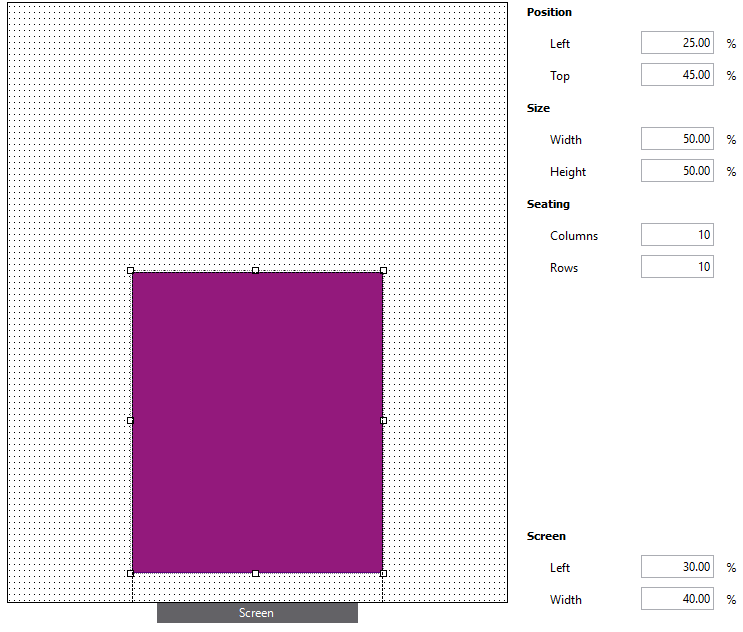
Areas
A screen can contain multiple areas. An area defines a block of seats, with each seat being of equal size, and displayed in rows and columns. An area is positioned within the boundary of a screen layout. For example, the following screen layout has 3 areas positioned horizontally adjacent to each other:
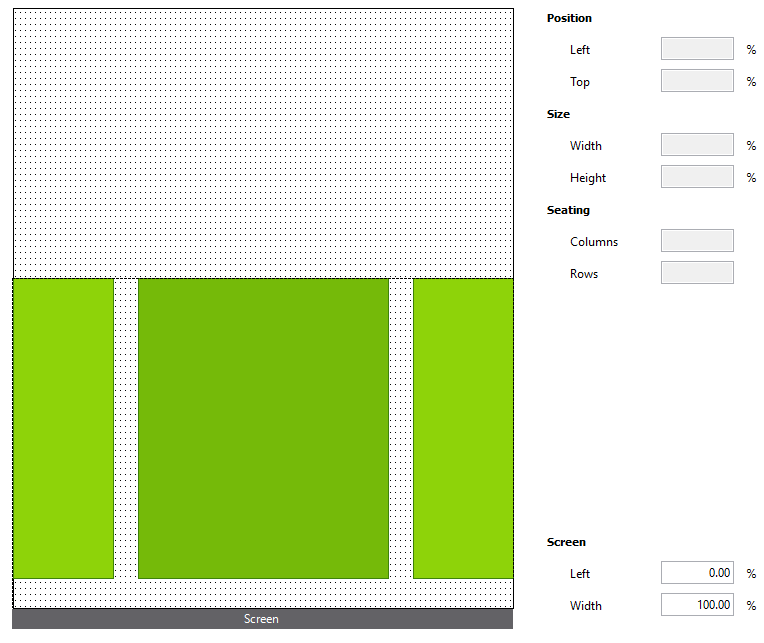
The positioning of each of these areas is represented in the areas object with the following properties (some properties have been removed for brevity):
{
"areas": [
{
"number": 1,
"columnCount": 10,
"rowCount": 10,
"left": 25,
"top": 45,
"width": 50,
"height": 50,
},
{
"number": 2,
"columnCount": 4,
"rowCount": 10,
"left": 0,
"top": 45,
"width": 20,
"height": 50,
},
{
"number": 3,
"columnCount": 4,
"rowCount": 10,
"left": 80,
"top": 45,
"width": 20,
"height": 50,
}
]
}
The left and top properties are used to position the area within the layout boundaries. The rowCount and columnCount define the grid size of the area. Not every grid cell has to contain a seat. A cell with no seat defined in the seats array property should be rendered as an empty space, and is one way in which aisles may be defined in a seat layout.
Some areas can be rendered larger than other areas, usually done to promote the purchasing of seats in that area. The width and height are used to define how large the total render area is, and therefore can be used to calculate the render size of the seats in that area. In the preceding JSON excerpt, all seats are equally sized (5x5).
Rows and Seats
Each area has rows, and each row contains a label for the row and an array of seats. For example:
{
"rows": [
{
"rowLabel": "M",
"seats": [
{
"position": {
"areaNumber": 1,
"rowIndex": 0,
"columnIndex": 6
},
"seatsInGroup": [],
"seatLabel": "11",
"status": 0,
"originalStatus": 0
},
{
"position": {
"areaNumber": 1,
"rowIndex": 0,
"columnIndex": 7
},
"seatsInGroup": [],
"seatLabel": "12",
"status": 0,
"originalStatus": 0
},
]
}
]
}
The JSON excerpt contains two seats: M11, and M12. These are positioned within the area using the rowIndex and columnIndex properties. The status field defines the seat type and its availability:
Seat Status Code | Description |
---|---|
0 | Available for selection |
1 | Sold |
2 | House Seat (commonly used to indicate a seat that is unavailable due to social distancing rules) |
3 | Wheelchair Seat |
4 | Reserved for this order |
5 | Unavailable (Due to seat being broken etc.) |
7 | Companion seat for wheelchair seat |
When a seat is selected by the customer, the seat’s status changes from one of the ‘available’ statuses (0, 3 or 7) to the reserved status (4). Querying the GET seats endpoint again, the originalStatus field will indicate what type of seat the reserved seat was (standard, wheelchair, or companion – 0, 3 or 7 respectively).
Seat Groups - Sofa Seats and Wheelchair Companion Seats
{
[
{
"rowLabel": "K",
"seats": [
{
"position": {
"areaNumber": 2,
"rowIndex": 0,
"columnIndex": 0
},
"seatsInGroup": [
{
"areaNumber": 2,
"rowIndex": 0,
"columnIndex": 0
},
{
"areaNumber": 2,
"rowIndex": 0,
"columnIndex": 1
}
],
"seatLabel": "1",
"status": 0,
"originalStatus": 0
},
{
"position": {
"areaNumber": 2,
"rowIndex": 0,
"columnIndex": 1
},
"seatsInGroup": [
{
"areaNumber": 2,
"rowIndex": 0,
"columnIndex": 0
},
{
"areaNumber": 2,
"rowIndex": 0,
"columnIndex": 1
}
],
"seatLabel": "2",
"status": 0,
"originalStatus": 0
},
]
}
]
}
Some seats have relationships with other seats that require them to be rendered differently; for example, sofa seats, or companion seats for wheelchair seats. These relationships are defined by the seatsInGroup property on a seat. The property lists which seats are in a group with the current seat. In the case of sofa seats, the left seat, middle seats and right seat can be determined based on the row and column Index. For wheelchair and companion seats, the seat group type can be determined by the seat status. The preceding code sample shows how each of these seat groups can be represented.
Seat Selection and Area Categories
A seat layout can contain areas for which only a certain category of tickets is applicable. These are distinguished by area categories, and are commonly used to create a different price band between premium seating and standard seating. The ticket type contains an area category code that indicates which seat area(s) the customer can select seats for, and each area belongs to an area category. The seat layout has an areaCategories property that indicates which how many seats can be selected in each area:
{
"areaCategories": [
{
"areaCategoryCode": "0000000000",
"seatsToAllocate": 1,
"seatsAllocatedCount": 1,
"seatsNotAllocatedCount": 0,
"selectedSeats": [
{
"areaNumber": 1,
"rowIndex": 3,
"columnIndex": 3
}
]
},
{
"areaCategoryCode": "0000000001",
"seatsToAllocate": 1,
"seatsAllocatedCount": 1,
"seatsNotAllocatedCount": 0,
"selectedSeats": [
{
"areaNumber": 2,
"rowIndex": 6,
"columnIndex": 9
}
]
}
]
}
When retrieving a seat layout, seats may have been automatically allocated for the customer. This is indicated by the seatsToAllocate and seatsAllocatedCount properties. In cases where the cinema ticketing system has not automatically allocated the customer’s seats the seatsNotAllocatedCount will be greater than zero.
Response Properties
Layout(root level object)
Response Property | Description |
---|---|
boundaryRight | The rightmost position of the seating areas within the screen layout boundary. This value is a 0-100 percentage of the screen layout boundary. |
boundaryLeft | The leftmost position of the seating areas within the screen layout boundary. This value is a 0-100 percentage of the screen layout boundary. |
boundaryTop | The topmost position of the seating areas within the screen layout boundary. This value is a 0-100 percentage of the screen layout boundary. |
screenStart | The leftmost position of the screen within the screen layout boundary. This value is a 0-100 percentage of the screen layout boundary. |
screenWidth | The width of the screen within the screen layout boundary. This value is a 0-100 percentage of the screen layout boundary. |
areaCategories | List of Area Categories in the screen layout |
areas | List of Areas in the screen layout |
Area Categories
Response Propety | Description |
---|---|
areaCategoryCode | The ID of the area category. See Seat Selection and Area Categories for details. |
seatsToAllocate | The total number of seats that must be allocated for the area category. |
seatsAllocatedCount | The number of seats that have been automatically allocated. |
seatsNotAllocatedCount | The number of seats that have not been allocated. |
selectedSeats | List of Seat Positions of the seats that have been allocated. |
Seat Position
Response Property | Description |
---|---|
areaNumber | Identifies which area the seat belongs to. |
rowIndex | The row within the area the seat belongs to. |
columnIndex | The column within the area the seat belongs to. |
Area
Response Property | Description |
---|---|
number | Uniquely identifies the area within this seat layout. |
areaCategoryCode | The area category code this area belongs to. See Seat Selection and Area Categories for details. |
columnCount | The total number of seats within this area. |
rowCount | The number of columns of seats in the area. |
description | A textual description of the area; for example, Premium, Stalls. |
left | The leftmost position of the area within the screen layout boundary. This value is a 0-100 percentage of the screen layout boundary. |
top | The topmost position of the area within the screen layout boundary. This value is a 0-100 percentage of the screen layout boundary. |
width | The width of the area. This value is a 0-100 percentage of the screen layout boundary. |
height | The height of the area. This value is a 0-100 percentage of the screen layout boundary. |
rows | List of Rows in the area. |
Row
Response Property | Description |
---|---|
rowLabel | The name of the row. |
seats | List of Seats in the row. |
Seat
Response Property | Description |
---|---|
position | The Seat’s Position. |
seatsInGroup | List of Seat Positions that make up the seat group. |
seatLabel | The name of the seat. Commonly combined with the row label to give a description of the seat; for example, “A12”. |
status | The seat’s status. See Rows and Seats for the list of possible seat statuses. |
originalStatus | Indicates a seat's status prior to it being reserved. See Rows and Seats for details. |
Updated over 4 years ago